This post is the first entry of a multi-part series leading toward dynamically targeting endpoints for administrative activity by leveraging questions and actions issued via the Tanium API. Each new post will introduce some concepts of increasing difficulty and is intended for those with intermediate PowerShell and Tanium knowledge that want to begin leveraging the Tanium API. The plan for the series is as follows:
- Starting the Conversation – Asking basic questions via the Tanium API
- Taking Action – Issuing basic Actions via the Tanium API
- Going to the Max – Writing basic automation to dynamically target an online audience with consideration for hard maximums
- Bringing it Home – A real-world example of how this capability can be utilized to supplement most environments in simple, effective ways
Starting up a conversation in an unfamiliar setting can be intimidating. The only way we can improve is to practice so let’s get to work.
Introductions
TanREST PowerShell Module
This series will rely upon access to the Tanium TanREST module for PowerShell. This software is not publicly distributed so you will need to contact your TAM in order to acquire the content required to proceed with the series.
Importing TanREST
The TanREST module can either be automatically imported using PowerShell profiles or it can be manually imported via the Import-Module cmdlet. Great articles have already been written about them in addition to the internal documentation that can be accessed by running Get-Help about_profiles in a PowerShell session.
Your TAM will provide you with a zip archive. In my experience, this has been named TanRest-master.zip. In order to import the TanRest module, perform the following:
- Extract the archive to a location that you can remember. For our purposes, let’s assume you extracted the archive to C:\Users\[yourUsernameHere]\Documents\TanREST-master.
- Start an Admin PowerShell session
- Run the following command: Import-Module “C:\Users\[yourUsernameHere]\Documents\TanREST-master\TanRest”
- Run the following command to validate that you now have access to TanRest functions: Get-Command -Module TanRest
Finding the Syntax
One of the quickest ways to get off track in this endeavor is to be imprecise with the question structure. The biggest shift in the way that we are trying to interact with Tanium is that we are avoiding one of earliest and most valuable tools one learns while initially learning about the platform; the natural language parser. We can afford to be a little sloppy during our day-to-day question building because the natural language parser can usually clean up the subtle syntactical mistakes that we make. We need to be precise every time we intend to ask questions via the API. Precision ensures that we target what we truly intend to target with automation we will use later in the series.
We are going to identify a cohort of endpoints that meet all of these basic conditions:
- Windows OS
- Virtualized
- Workstations
We will use the Question Builder in Tanium Interact to get the exact question we are looking for. Again, precision matters here.
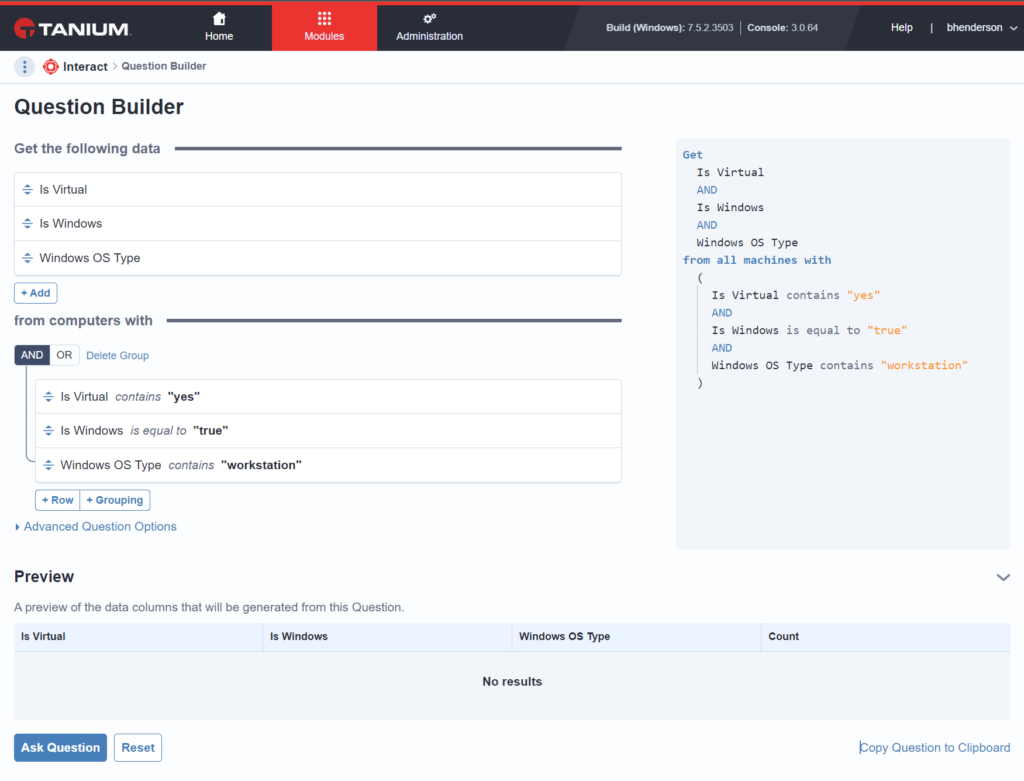
The example above was structured to demonstrate two points:
- Exploratory questions asked via the Question Builder are perfectly fine if you are ever unsure of the results of a given sensor and want to confirm that your targeting filter logic under the from computers with ___________ section is correct. You may also refer to the sensor browser to confirm the potential outputs of a given sensor.
- Boolean consistency is not guaranteed between sensors. There is variability even among sensors that provide Boolean output so you should not assume what they are looking for because of the potential for major mistakes.
- Example: If you were using negative logic with the Is Windows sensor and set your filtering logic to Is Windows is not equal to No (The negative logic equivalent to Is Windows equals Yes), the results will include non-Windows operating systems because the Is Windows sensor returns true or false rather than yes or no and a Linux OS that responds with a false will not be excluded by that mistake in your filtering question. I would avoid using negative logic because it introduces an unnecessary layer of ambiguity in circumstances like these.

The results are what we desired so we can safely convert the exploratory question into the targeting question below:
Get Online from all machines with ( Is Virtual contains yes and ( Is Windows equals true and Windows OS Type contains workstation ) )
Asking the Question
We have done the following at this point:
- Acquired the TanRest-master.zip file from a Tanium TAM
- Extracted the zip archive to a local directory
- Imported the TanREST module into a PowerShell session
- Determined the structure of the basic question that we want to ask via the API
I uploaded a companion script to Github that demonstrates the next steps of the process. Code heavy posts make for a poor read so I have opted to leave most of that to the companion script. There are some details of the script that I do believe warrant a bit of discussion:
- I leverage a PSCustomObject to hold critical information. A PSCustomObject is basically a fancy hash table with key-value pairs that are represented as properties of an object. The reasoning for this will become apparent in subsequent posts but the simple explanation is that I prefer a clean, well-defined object with subordinate properties than a lot of independently declared/defined variables.
- There is esoteric language exposed in the code whose meaning isn’t obvious at first glance. Take this if header for example: if($questionObject.parsed.from_canonical_text -eq 1)
- $questionObject is the PSCustomObject defined in the script
- parsed, a property of $questionObject, is the Tanium platform’s interpretation of the question that we pass to the natural language parser via TanREST
- from_canonical_text, a subproperty of the parsed property of $questionObject, is an indication of the parser’s ability to decipher your question. A value of 1 indicates that the parser “understood” your question and found no ambiguity in the structure of the question that was provided.
- A question submitted via the API must go through three separate functions:
- New-TaniumCoreParseQuestion
- Parses the defined question to ensure that the natural language parser understands what you have provided
- New-TaniumCoreQuestion
- Submits the parsed question to the Tanium platform which will in turn collect that information from the client fleet
- Get-TaniumCoreQuestionResult
- Returns the results of the aforementioned question as an object that can be acted upon with further PowerShell scripting
- New-TaniumCoreParseQuestion

The screenshot above is the contents of your $questionObject should look like after you have followed the companion script.
This is a lot of material to cover so do not feel discouraged if every element of the content doesn’t “click” right away. As always, feel free to comment if you have any questions or feedback.
Disclaimer: Any code made available on this site is free to use at your own discretion but it is provided without any explicit or implied guarantees of support, reliability, or functionality. I accept no responsibility in the event that the code, in its original form or any derivative versions thereafter, malfunctions or causes problems . Anything from this site that you decide to work with should be tested thoroughly in development environments in collaboration with your Technical Account Manager (TAM) until such time that you, the responsible party, decides that you are satisfied with its outcomes.
3 replies on “Conversing with TanREST Part I: Starting the Conversation”
[…] Starting the Conversation – Asking basic questions via the Tanium API […]
[…] Starting the Conversation – Asking basic questions via the Tanium API […]
Excellent work. Thank you for creating such a well structured post.